Requirements
ImageMagick – ready-to-run binary distribution or as source code to create, edit, compose, or convert digital images (Website)
- Use sudo apt install on Ubuntu
- Use sudo yum install on CentOS
Intervention Image – PHP image handling and manipulation library (Website)
- composer require intervention/image
<?php require './vendor/autoload.php'; use Intervention\Image\ImageManagerStatic as Image; $img = Image::make('source-image.jpg'); $image_width = 300; // Apply a smart crop $img->fit($image_width); // create empty canvas with transparent background $canvas = Image::canvas($image_width, $image_width); // draw a black circle on it $canvas->circle($image_width, $image_width/2, $image_width/2, function ($draw) { $draw->background('#000000'); }); // Mask your image with the shape $img->mask($canvas->encode('png', 75), true); // 75 is the image compression ratio // Response with the image or you can as well do whatever you like with it. echo $img->response('png');
Sample Images
Source Image
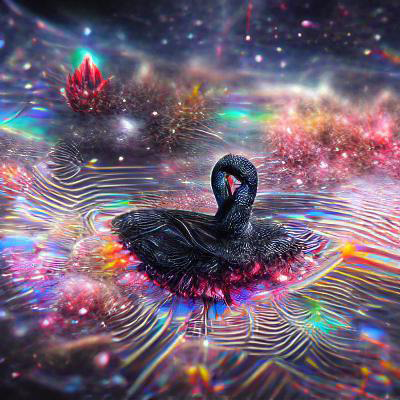
Source Image (e.g. 400*400 Image In JPG)
Result Image

Result Image: 300*300 Rounded Corner Image In PNG
Additional Comments
It seems that the edge of the rounded corner image (300*300 Rounded Corner Image In PNG) is not that perfect (compared to what the Browser CSS border-radius can do), it contains some zigzag shape on the border outline. We can only resize it to a smaller size to make it look less obvious.
$resized_width = $image_width * 0.6; $resized_img = Image::make($img->response('png')); $resized_img->resize($resized_width, $resized_width); echo $resized_img->response('png');

Resized Image: 180*180 Rounded Corner Image In PNG
Related Keywords: Developer, How-to, Solved, PHP image manipulation, php image border radius, rounded corners images